Yesterday I wrote a post about How to specific a port to device with iOSOpenDev. It's more like a tutor due to lacking of the process of analysis. So I decide to write about the process how did I analyze it so you can add this feature on yourselves when iOSOpenDev updates someday.
I'll keep this post as simple as I can, but I'll assume you've already had some basic knowledge.
We can see the `Run Script` right under Xcode's `Build Phases` section.
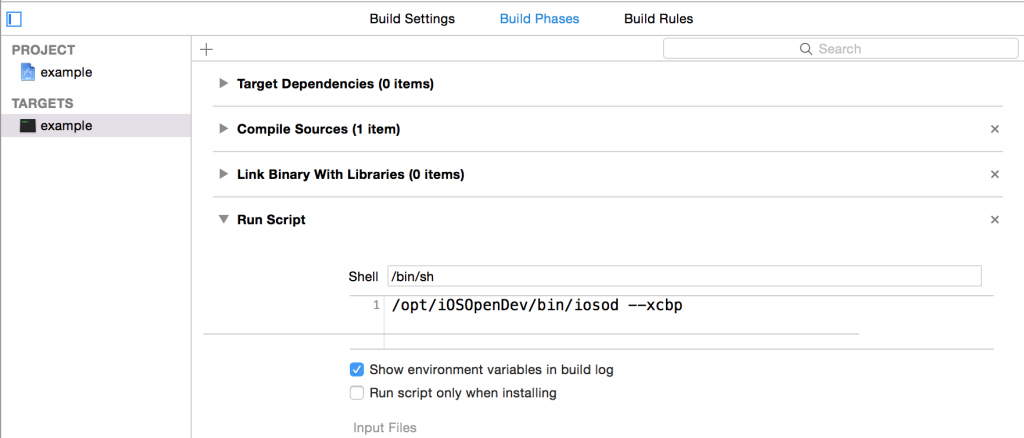
It writes `/opt/iOSOpenDev/bin/iosod --xcbp`.
So let's check on /opt/iOSOpenDev/bin/iosod.
And `file` suggests that this is a shell script.
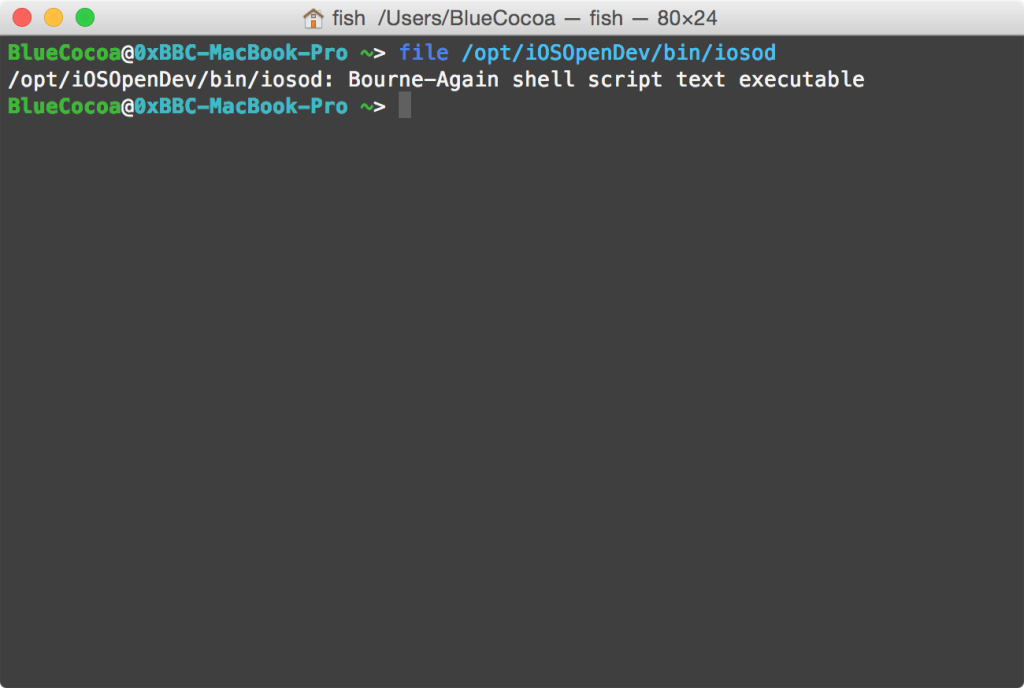
Great, at least we don't need to perform a reverse engineering on it :)
At this point, I assume you to know that iOSOpenDev uses SSH and SCP to connect to and copy files to iOS devices.
Then open it in your favorite text editor and search the first keyword, 'ssh'.
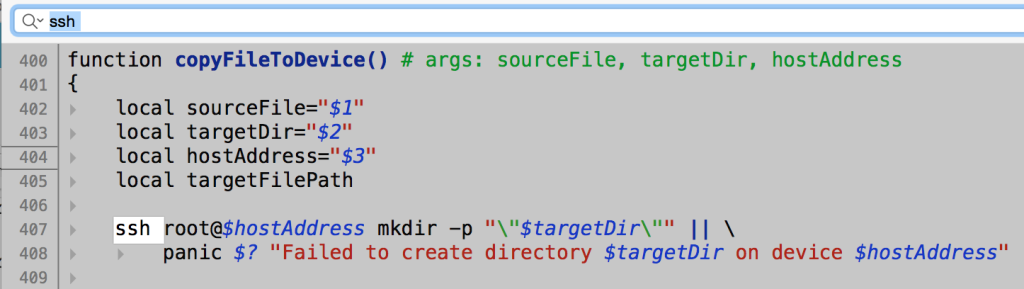
You'll found a lot matched lines. But I will only take some of them as examples because the modifications we'll make to them are exactly the same with examples.
To specific a port,
- SSH
ssh -p port user@machine
- SCP
scp-P port paths_of_files user@machine:path
In order to make it more flexible, I want to use a variable just like iOSOpenDev do.
Look at this function,
{
local sourceFile="$1"
local targetDir="$2"
local hostAddress="$3"
local targetFilePath
...
Let's add a variable named hostPort.
{
local sourceFile="$1"
local targetDir="$2"
local hostAddress="$3"
local hostPort="$4"
local targetFilePath
...
Then, let's replace `ssh root@$hostAddress` with `ssh -p $hostPort root@$hostAddress`, and replace `scp "$sourceFile" root@$hostAddress` with `scp -P $hostPort"$sourceFile" root@$hostAddress` first.
After the modification, the function will look like in this way,
{
local sourceFile="$1"
local targetDir="$2"
local hostAddress="$3"
local hostPort="$4"
local targetFilePath
ssh -p $hostPort root@$hostAddress mkdir -p ""$targetDir""||
panic $?"Failed to create directory $targetDir on device $hostAddress" targetFilePath="$targetDir/`basename "$sourceFile"`" ||
panic $?"Failed to build target file path"
ssh -p $hostPort root@$hostAddress rm -f ""$targetFilePath""||
panic $?"Failed to remove existing file $targetFilePath on device $hostAddress" scp -P $hostPort"$sourceFile" root@$hostAddress:""$targetFilePath""||
panic $?"Failed to copy file $sourceFile to device $hostAddress at directory $targetDir"
}
Now, do the same job to other functions if they called `ssh` or `scp`.
When you modify managePackageOnDevice(), you'll find that managePackageOnDevice() checks hostAddress value
requireExportedVariable "iOSOpenDevDevice"
hostAddress="$iOSOpenDevDevice" [[ $hostAddress != "" ]] ||
panic 1 "Host address not provided and environment variable iOSOpenDevDevice is not set or is empty"
fi
Alright, we need to check our variable, too. And let's use `iOSOpenDevDevicePort` as its name in Xcode.
requireExportedVariable "iOSOpenDevDevicePort"
hostPort="$iOSOpenDevDevicePort"
[[ $hostPort != "" ]] || $hostPort ="22"
# set 22 as default port
fi
So, the final version of copyFileToDevice() is,
{
local sourceFile="$1"
local targetDir="$2"
local hostAddress="$3"
local hostPort="$4"
local targetFilePath
if [[ $hostPort == "" ]]; then
requireExportedVariable "iOSOpenDevDevicePort"
hostPort="$iOSOpenDevDevicePort"
[[ $hostPort != "" ]] || $hostPort ="22"
# set 22 as default port
fi
ssh -p $hostPort root@$hostAddress mkdir -p ""$targetDir""||
panic $?"Failed to create directory $targetDir on device $hostAddress" targetFilePath="$targetDir/`basename "$sourceFile"`" ||
panic $?"Failed to build target file path"
ssh -p $hostPort root@$hostAddress rm -f ""$targetFilePath""||
panic $?"Failed to remove existing file $targetFilePath on device $hostAddress" scp -P $hostPort"$sourceFile" root@$hostAddress:""$targetFilePath""||
panic $?"Failed to copy file $sourceFile to device $hostAddress at directory $targetDir"
}
Okay, we have changed those functions, and we have to change the params when involve those functions.
Search those functions' name, and add "$hostPort" to the proper position.
e.g.
copyFileToDevice "$packageFileOrName""$devicePackagesDir""$hostAddress""$hostPort"
And then, modify the optarg.
There are also several optargs to modify. I'll take one of them as example.
The original optarg is

Now, we need to add a param, and we set the short name as 'p'
(If you use optarg in C, I believe you know this clearly.)

Finally, append "$Opt_p" to each "$Opt_h"
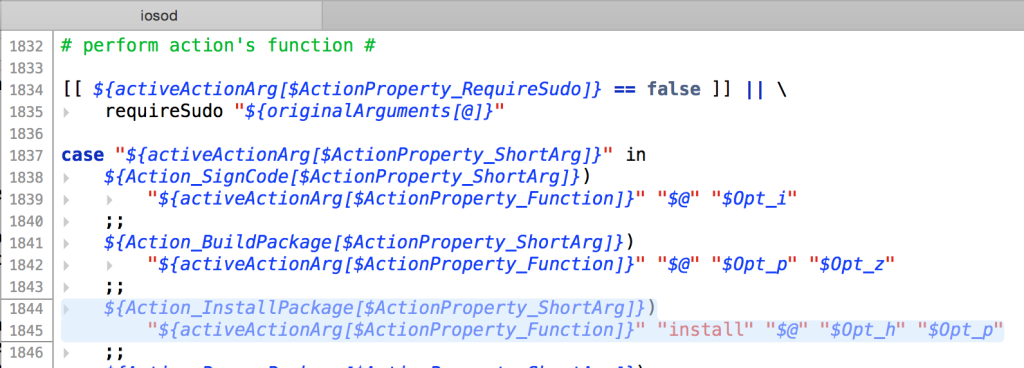
Save and replace the original iosod file.
And yes, you need to modify one of the template files, `/opt/iOSOpenDev/templates/Base.xctemplate/TemplateInfo.plist`.
Open it in Xcode, add one row named `iOSOpenDevDevicePort` under `Targets` -> `Item 0` -> `SharedSettings`. The default value of iOSOpenDevDevicePort is 22.
Finally,
vim ~/.bash_profile
add export iOSOpenDevDevicePort=22 right under export iOSOpenDevDevice
P.S
- For tweaks already exist
Find its xcodeproj file, `show contents`. Then edit project.pbxproj inside and search `iOSOpenDevDevice`.
Add
iOSOpenDevDevicePort = "22";
under iOSOpenDevDevice.
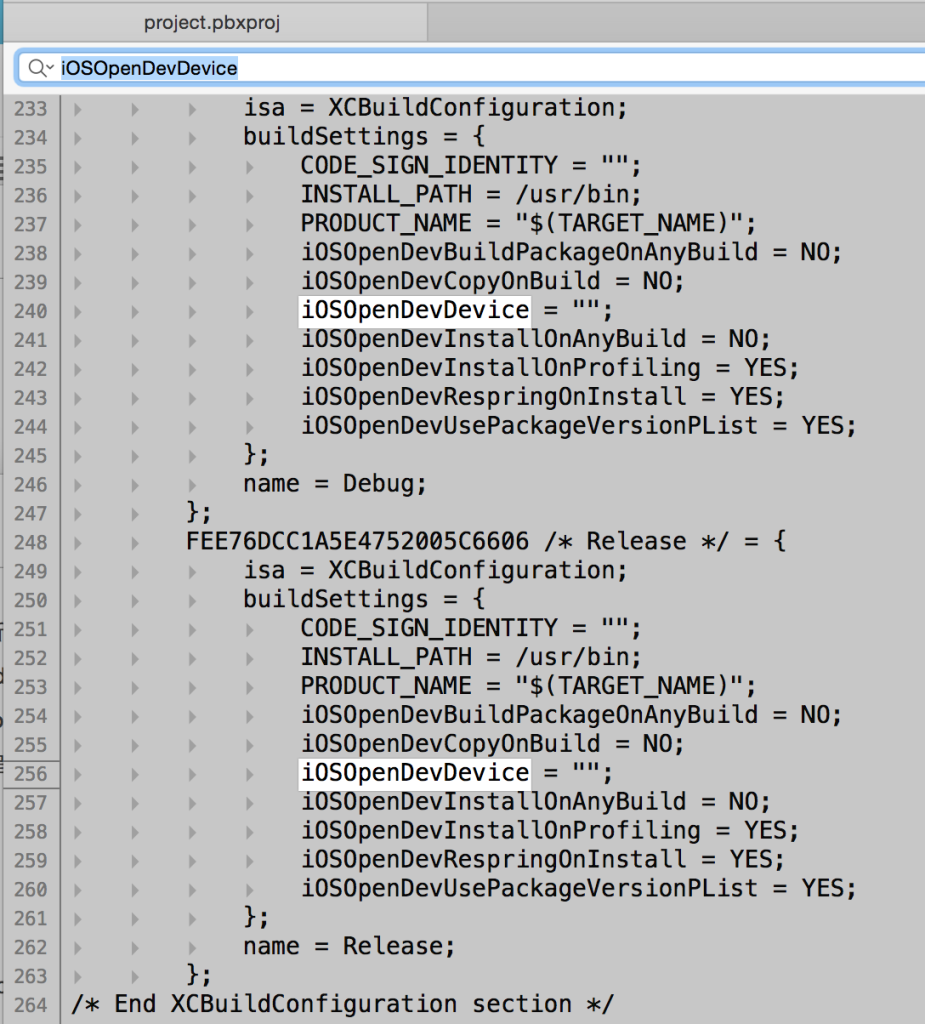
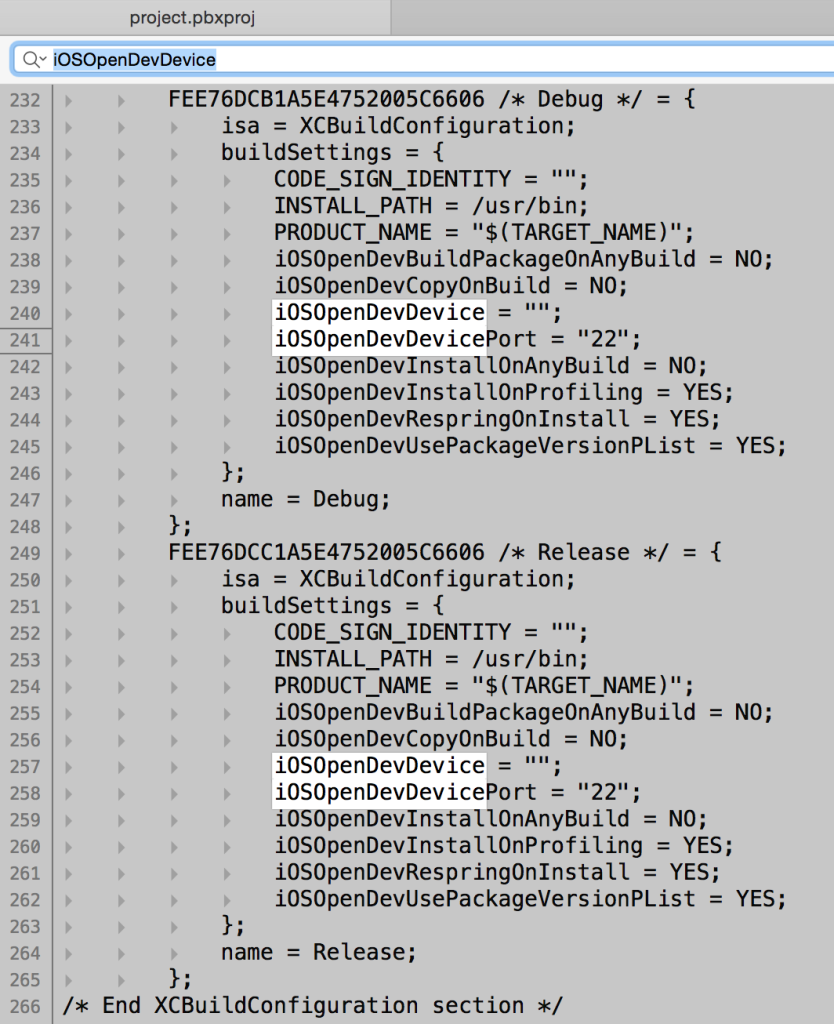